- Programming
- 0 (Registered)
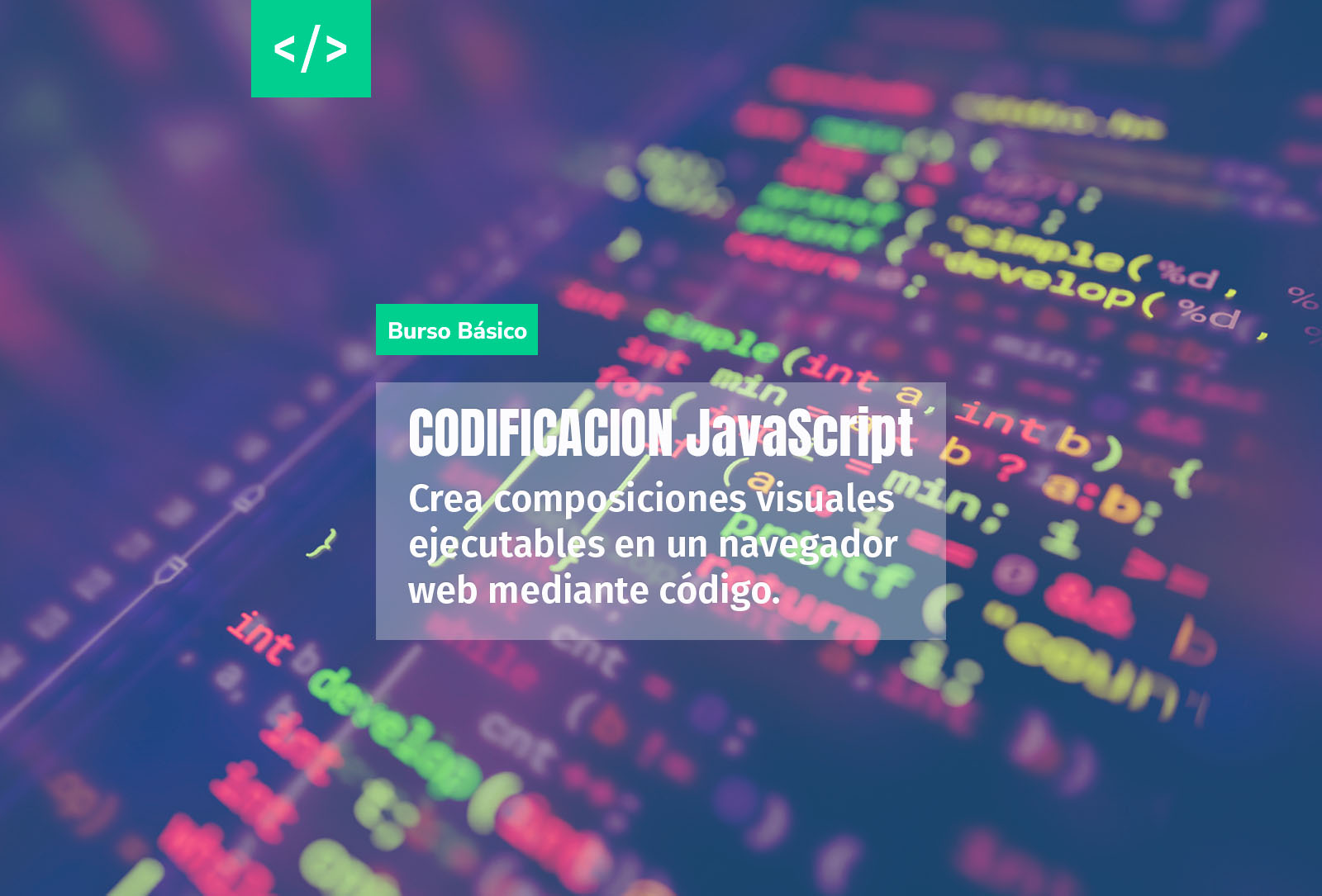
JavaScript Intermediate Level
Course Description
This intermediate-level JavaScript course is designed for developers who already have a basic understanding of the language and wish to take their skills to the next level. Throughout the course, students will delve into more advanced concepts and learn how to build more robust and efficient web applications.
We will explore topics such as object-oriented programming (OOP) in JavaScript, advanced event handling, DOM manipulation beyond the basics, and how to effectively work with APIs and promises. The course will also cover modern development techniques with ES6+ and how to apply best practices in writing clean and maintainable code.
Course Objectives
By the end of this course, students will be able to:
- Apply principles of object-oriented programming in JavaScript to create modular and reusable code.
- Utilize advanced DOM manipulation and event handling techniques to enhance interactivity in web applications.
- Work with external APIs and manage asynchronous requests using promises and async/await.
- Implement advanced data structures and algorithms to optimize application performance.
- Understand and apply modern JavaScript (ES6+) features, such as modules, destructuring, and arrow functions.
- Develop more complex web applications using popular frameworks and libraries.
Course Content
- Object-Oriented Programming in JavaScript
- Key OOP concepts: Classes, inheritance, encapsulation, and polymorphism.
- Implementation of design patterns in JavaScript.
- Advanced DOM Handling
- Advanced DOM manipulation: Creating and modifying nodes dynamically.
- Event delegation and custom events.
- Working with APIs and Promises
- Handling asynchronous requests with fetch and promises.
- Using async/await to simplify asynchronous code.
- Modern JavaScript Techniques (ES6+)
- Introduction to ES6+: Let, const, and arrow functions.
- JavaScript modules: Importing and exporting functions.
- Destructuring arrays and objects.
- Algorithms and Data Structures
- Implementation of common algorithms: Searching, sorting, and recursion.
- Using advanced data structures such as stacks, queues, and trees.
- Developing Complex Applications
- Introduction to frameworks and libraries: React, Vue.js, Angular.
- Developing a complete application using best practices and design patterns.
Projects and Evaluation
- Project 1: Create an interactive web application using advanced DOM manipulation and event techniques.
- Project 2: Develop a web service that consumes multiple APIs and displays dynamic data, implementing async/await.
- Final Project: Build a complex web application using a framework or library, applying all the concepts learned in the course.
This course is ideal for those who already have basic experience with JavaScript and are ready to take on more complex challenges in web development. With a combination of theory and practice, students will be prepared to build modern and scalable web applications.